Simply Visual Basic 2010: An App-Driven Approach, International Edition, 4th edition
Published by Pearson (July 19, 2012) © 2012
- Harvey M. Deitel Deitel & Associates, Inc.
- Paul Deitel Deitel & Associates, Inc.
- Abbey Deitel Deitel & Associates, Inc.
eTextbook
- Easy-to-use search and navigation
- Add notes and highlights
- Search by keyword or page
- A print text (hardcover or paperback)
- Free shipping
For introductory courses in Visual Basic Programming, offered in departments of Information Technology, Computer Science or Business.
Merging the concept of a lab manual with that of a conventional textbook, the Deitels have crafted an innovative approach that enables students to learn programming while having a mentor-like book by their side. This best-seller blends the DeitelTM signature Live-CodeTM Approach with their Application-DrivenTM methodology. Students learn programming and Visual Basic by working through a set of applications. Each tutorial builds upon previously learned concepts while learning new ones. An abundance of self assessment exercises are available at the end of most chapters to reinforce key ideas.
This approach makes it possible to cover a wealth of programming constructs within the Visual Basic 2010 environment.
View the Deitel Buzz online to learn more about the newest publications from the Deitels.
Signature “Live CodeTM Approach” – Language features are presented in the context of a wide variety of complete working programs.
– Focuses on complete working programs.
– Enables students to confirm that programs run as expected.
– Students can download the code from the book's Companion Website (www.pearsonhighered.com/deitel) or from the authors' website (www.deitel.com).
Outstanding, consistent and applied pedagogy:
– Icons throughout identify Software Engineering Observations; Good Programming Practices; Common Programming Errors; Portability Tips; Performance Tips, Testing and Debugging Tips, and Look-and-Feel Observations.
– Full-color presentation, including syntax coloring, code highlighting, callouts and extensive comments
– Skills summaries and helpful self-review multiple-choice questions and answers for immediate feedback after each section
Early immersion in visual programming techniques and modifying Visual Basic .NET GUIs gives students a foundation for designing GUIs, concepts that they will apply throughout the book as they are taught core programming concepts. Special sections on GUI Design Guidelines and on Controls, Events, Properties & Methods.
Careful introduction to creating objects – Students begin to create objects and classes later in the book, while they use objects from .NET’s Framework Class Library throughout the book.
Extensive set of interesting exercises and substantial projects – Enable students to apply what they've learned in each chapter.
- Updated to Visual Basic 2010, the Visual Studio 2010 IDE and .NET 4.
- ASP treatment updated to ASP.NET 4.
- The hundreds of screenshots have been replaced by easier to read code listings with line numbers, syntax shading and code highlighting.
- Focus on business and personal utility examples.
- Shifted the thinking from “application development” to “app development”–many of the apps in Simply Visual Basic 2010 inspired the apps in the Deitel Developer Series books, iPhone for Programmers and Android for Programmers.
- The authors will test all of the examples and exercises on Windows 8 when it is released and post any updates on the www.deitel.com/books/simplyvb2010/.
- Integrated visual programming with Windows Forms GUI throughout, LINQ with generic collections, LINQ with database, database with Microsoft SQL Server Express, Windows Presentation Foundation (WPF) GUI and graphics, GDI+ graphics.
- App-driven, live-code approach. All of the concepts and exercises are presented in the context of real-world apps.
- Integrated debugging.
- Companion Website includes video introduction to the Visual Basic 2010 Express IDE, Before You Begin video and other resources.
- The book is supported by a growing list of online resource centers at www.deitel.com/ResourceCenters.html.
- Instructor ancillaries include PowerPoint Slides, Instructor’s Solutions Manual and Test Item File.
1 Test-Driving a Painter App 1
Introducing Computers, the Internet and Visual Basic 1
1.1 Computing in Business, Industry and Research 1
1.2 Hardware and Software 5
1.3 Data Hierarchy 6
1.4 Computer Organization 8
1.5 Machine Languages, Assembly Languages and High-Level Languages 10
1.6 Object Technology 11
1.7 Microsoft’s Windows® Operating System 13
1.8 Programming Languages 13
1.9 Visual Basic 14
1.10 The Internet and the World Wide Web 15
1.11 Microsoft .NET 16
1.12 Web 2.0: Going Social 17
1.13 Test-Driving the Visual Basic Advanced Painter App 21
1.14 Web Resources 24
1.15 Wrap-Up 24
2 Welcome App 37
Introducing the Visual Basic 2010 Express IDE 37
2.1 Test-Driving the Welcome App 37
2.2 Overview of the Visual Studio 2010 IDE 38
2.3 Creating a Project for the Welcome App 40
2.4 Menu Bar and Toolbar 45
2.5 Navigating the Visual Studio IDE 47
Solution Explorer 48
Toolbox 49
Properties Window 49
2.6 Using Help 51
2.7 Saving and Closing Projects in Visual Basic 53
2.8 Web Resources 53
2.9 Wrap-Up 54
3 Welcome App 65
Introduction to Visual App Development 65
3.1 Test-Driving the Welcome App 65
3.2 Constructing the Welcome App 68
3.3 Objects Used in the App 78
3.4 Wrap-Up 78
4 Designing the Inventory App 106
Introducing TextBoxes and Buttons 106
4.1 Test-Driving the Inventory App 106
4.2 Constructing the Inventory App 108
4.3 Adding Labels to the Inventory App 112
4.4 Adding TextBoxes and a Button to the Form 115
4.5 Wrap-Up 118
5 Completing the Inventory App 135
Introducing Programming 135
5.1 Test-Driving the Inventory App 135
5.2 Introduction to Visual Basic Program Code 136
5.3 Inserting an Event Handler 139
5.4 Performing a Calculation and Displaying the Result 143
5.5 Using the IDE to Eliminate Compilation Errors 146
5.6 Wrap-Up 149
6 Enhancing the Inventory App 163
Introducing Variables, Memory Concepts and Arithmetic 163
6.1 Test-Driving the Enhanced Inventory App 163
6.2 Variables 164
6.3 Handling the TextChanged Event 167
6.4 Memory Concepts 169
6.5 Arithmetic 170
6.6 Using the Debugger: Breakpoints 172
6.7 Wrap-Up 175
7 Wage Calculator App 190
Introducing Algorithms, Pseudocode and Program Control 190
7.1 Test-Driving the Wage Calculator App 190
7.2 Algorithms 191
7.3 Pseudocode 192
7.4 Control Statements 193
7.5 If…Then Selection Statement 195
7.6 If…Then…Else Selection Statement and Conditional If Expressions 197
7.7 Constructing the Wage Calculator App 200
7.8 Assignment Operators 204
7.9 Formatting Text 205
7.10 Using the Debugger: The Watch Window 207
7.11 Wrap-Up 210
8 Dental Payment App 225
Introducing CheckBoxes and Message Dialogs 225
8.1 Test-Driving the Dental Payment App 225
8.2 Designing the Dental Payment App 228
8.3 Using CheckBoxes 229
8.4 Using a Dialog to Display a Message 231
8.5 Logical Operators 235
Using AndAlso 235
Using OrElse 236
Short-Circuit Evaluation 237
Using Xor 237
Using Not 237
8.6 Designer-Generated Code 240
8.7 Wrap-Up 241
9 Car Payment Calculator App 256
Introducing the Do While…Loop and Do Until…Loop Repetition Statements 256
9.1 Test-Driving the Car Payment Calculator App 256
9.2 Do While…Loop Repetition Statement 258
9.3 Do Until…Loop Repetition Statement 260
9.4 Constructing the Car Payment Calculator App 262
9.5 Wrap-Up 269
10 Class Average App 282
Introducing the Do…Loop While and Do…Loop Until Repetition Statements 282
10.1 Test-Driving the Class Average App 282
10.2 Do…Loop While Repetitio
Need help? Get in touch
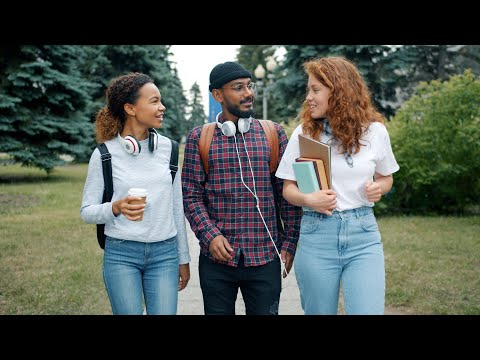
Pearson eTextbook: What’s on the inside just might surprise you
They say you can’t judge a book by its cover. It’s the same with your students. Meet each one right where they are with an engaging, interactive, personalized learning experience that goes beyond the textbook to fit any schedule, any budget, and any lifestyle.