Switch content of the page by the Role togglethe content would be changed according to the role
Sams Teach Yourself Java in 21 Days (Covers Java 11/12), 8th edition
Published by Sams Publishing (January 15, 2020) © 2020
- Rogers Cadenhead
eTextbook
$30.59
$35.99
Need help? Get in touch
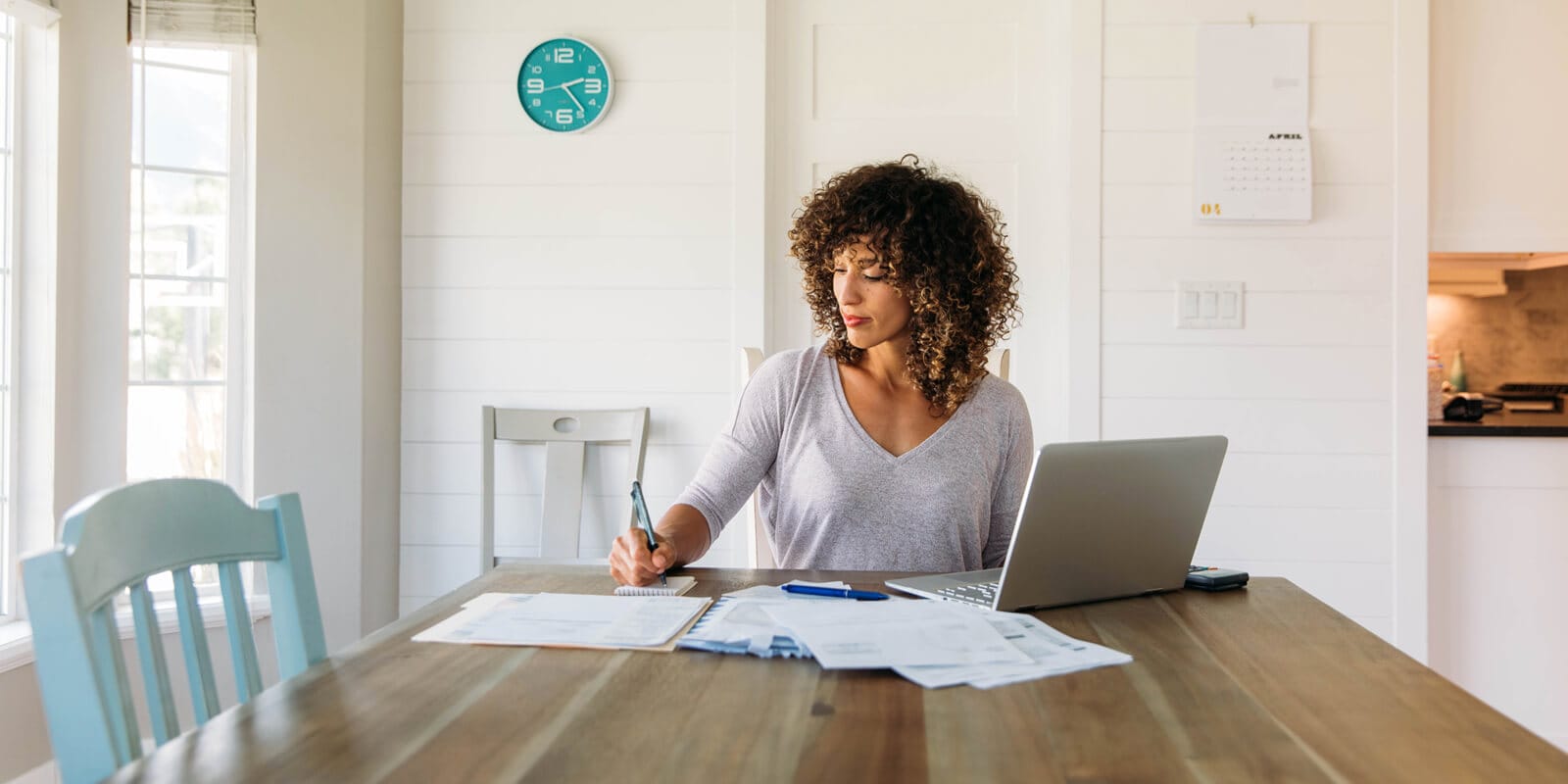
Digital Learning NOW
Extend your professional development and meet your students where they are with free weekly Digital Learning NOW webinars. Attend live, watch on-demand, or listen at your leisure to expand your teaching strategies. Earn digital professional development badges for attending a live session.